https://ckan.odpt.org/dataset/b_busstop-yokohamamunicipal/resource/da256719-0c39-48a7-a1f2-20354e18d529
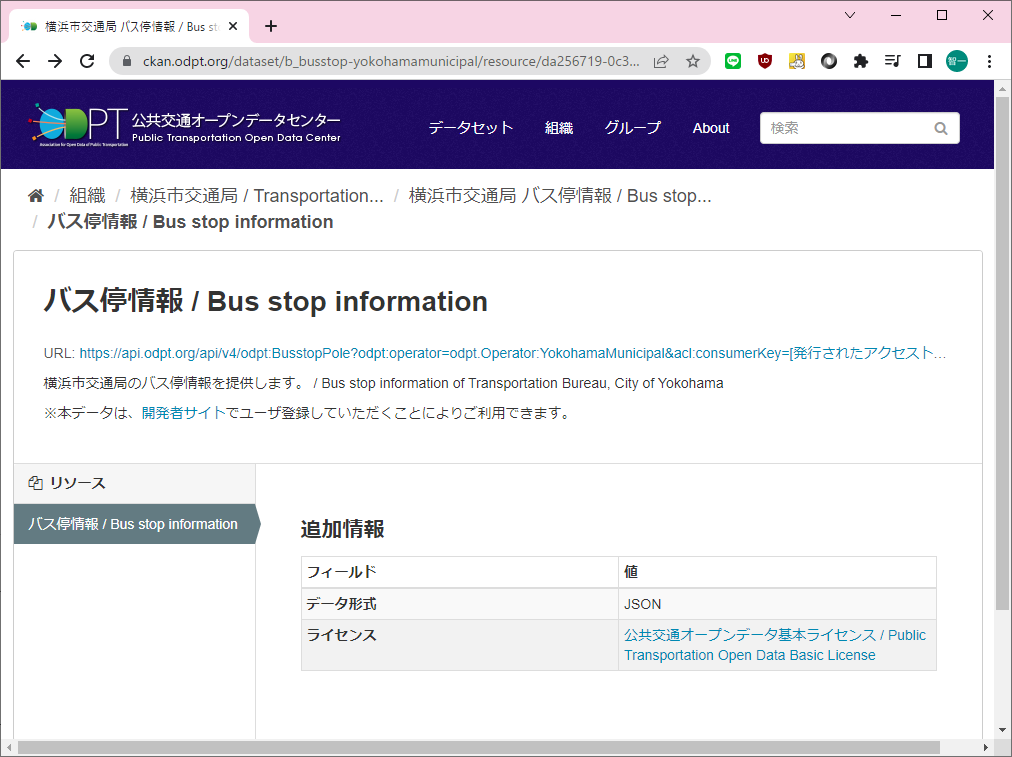
// stop_json.go
// 横浜市交通局 バス停情報 / Bus stop information of Transportation Bureau, City of Yokohama
// https://ckan.odpt.org/dataset/b_busstop-yokohamamunicipal
package main
import (
"encoding/json"
"fmt"
"io/ioutil"
"log"
)
type stopJSON struct {
Id string `json:"@id"`
Type string `json:"@type"`
Lng_Title struct {
En string `json:"en"`
Ja string `json:"ja"`
Ko string `json:"ko"`
Zh string `json:"zh"`
Ja_Hrkt string `json:"ja-Hrkt"`
} `json:"title"`
Date string `json:"dc:date"`
Lat float64 `json:"geo:lat"`
Long float64 `json:"geo:long"`
Context string `json:"@context"`
Title string `json:"dc:title"`
Kana string `json:"odpt:kana"`
SameAS string `json:"owl:sameAS"`
Operator []interface{} `json:"odpt:operator"`
BusroutePattern []interface{} `json:"odpt:busroutePattern"`
BusstopPoleNumber string `json:"odpt:busstopPoleNumber"`
// BusstopPoleTimetable
}
func main() {
// JSONファイルから読み取る場合
//file, err := ioutil.ReadFile("route.json")
//file, err := ioutil.ReadFile("odpt_BusroutePattern.json")
file, err := ioutil.ReadFile("odpt_BusstopPole.json")
if err != nil {
// エラー処理
}
/*
// Webアクセスで取得する場合
client := &http.Client{}
req, err := http.NewRequest("GET", "https://api.odpt.org/api/v4/odpt:BusstopPole?odpt:operator=odpt.Operator:YokohamaMunicipal&acl:consumerKey=f4954c3814b207512d8fe4bf10f79f0dc44050f1654f5781dc94c4991a574bf4", nil)
if err != nil {
log.Fatal(err)
}
resp, err := client.Do(req)
if err != nil {
log.Fatal(err)
}
defer resp.Body.Close()
if err != nil {
log.Fatal(err)
}
file, err := ioutil.ReadAll(resp.Body)
if err != nil {
log.Fatal(err)
}
*/
var data []stopJSON
err = json.Unmarshal(file, &data)
if err != nil {
fmt.Println("Unmarshal")
log.Fatal(err)
}
//fmt.Println(string(file))
// ループによる取得
for _, e := range data {
fmt.Println(e) // 全情報表情
stops := e.BusroutePattern
fmt.Println("len_stop", len(stops))
for _, stop := range stops {
fmt.Println(stop)
}
}
}