今月のコラムの原稿執筆の為、一次資料に遡っています。
Keyword;一体“孤独”の何が悪い? 『孤独は、1日タバコ15本分の害悪』―― その根拠はどこにある? イギリスの孤独担当相ダイアナ・バラン氏の発言 一次情報としては「死亡率の危険因子としての孤独と社会的孤立:メタ分析的レビュー(*)」という論文が出所らしい 死亡率に対する客観的および主観的な社会的孤立の相対的影響を調べること “社会的孤立(状態)”と”孤独(心理)”は、概念的に異なる(Coyle & Dugan, 2012; Perissinotto & Covinsky, 2014) “孤立”、“孤独”が招く、驚くべき死亡率の上昇 “たばこ15本” この死亡率を、喫煙による寿命の短縮(5.5分/1本→1.4時間/日)に
当てはめたのだろう(推測)
死亡率の危険因子としての孤独感および社会的孤立感。メタ分析的レビュー
------
Abstract
概要
Actual and perceived social isolation are both associated with increased risk for early mortality. In this meta-analytic review, our objective is to establish the overall and relative magnitude of social isolation and loneliness and to examine possible moderators. We conducted a literature search of studies (January 1980 to February 2014) using MEDLINE, CINAHL, PsycINFO, Social Work Abstracts, and Google Scholar. The included studies provided quantitative data on mortality as affected by loneliness, social isolation, or living alone. Across studies in which several possible confounds were statistically controlled for, the weighted average effect sizes were as follows: social isolation odds ratio (OR) = 1.29, loneliness OR = 1.26, and living alone OR = 1.32, corresponding to an average of 29%, 26%, and 32% increased likelihood of mortality, respectively. We found no differences between measures of objective and subjective social isolation. Results remain consistent across gender, length of follow-up, and world region, but initial health status has an influence on the findings. Results also differ across participant age, with social deficits being more predictive of death in samples with an average age younger than 65 years. Overall, the influence of both objective and subjective social isolation on risk for mortality is comparable with well-established risk factors for mortality.
------
実際の社会的孤立と認識された社会的孤立は、ともに早期死亡のリスク上昇と関連している。このメタ分析レビューでは、社会的孤立と孤独の全体的および相対的な大きさを確定し、可能なモデレーターを検討することを目的としている。MEDLINE、CINAHL、PsycINFO、Social Work Abstracts、Google Scholarを用いて、研究の文献検索(1980年1月~2014年2月)を行った。対象とした研究は、孤独感、社会的孤立、一人暮らしが影響する死亡率に関する定量的データを提供していた。いくつかの可能性のある交絡因子が統計的にコントロールされた研究全体で、加重平均効果量は以下の通りであった:社会的孤立のオッズ比(OR)=1.29、孤独のOR=1.26、一人暮らしのOR=1.32、これはそれぞれ平均29%、26%、32%の死亡率の上昇に対応している。客観的な社会的孤立と主観的な社会的孤立の測定値に差は見られなかった。結果は、性別、追跡期間、地域によって一貫しているが、初期の健康状態が結果に影響を及ぼしている。結果は参加者の年齢によっても異なり、平均年齢が65歳未満のサンプルでは、社会的欠陥が死亡をより予測することがわかった。全体として、死亡リスクに対する客観的および主観的な社会的孤立の影響は、死亡に対する確立された危険因子と同等である。
------
Keywords
social isolation, loneliness, mortality
------
キーワード
社会的孤立、孤独、死亡率
------
Several lifestyle and environmental factors are risk factors for early mortality, including smoking, sedentary lifestyle, and air pollution. However, in the scientific literature, much less attention has been given to social factors demonstrated to have equivalent or greater influence on mortality risk (Holt-Lunstad, Smith, & Layton, 2010). Being socially connected is not only influential for psychological and emotional well-being but it also has a significant and positive influence on physical well-being (Uchino, 2006) and overall longevity (Holt-Lunstad et al., 2010; House, Landis, & Umberson, 1988; Shor, Roelfs, & Yogev, 2013). A lack of social connections has also been linked to detrimental health outcomes in previous research. Although the broader protective effect of social relationships is known, in this meta-analytic review, we aim to narrow researchers’ understanding of the evidence in support of increased risk associated with social deficits. Specifically, researchers have assumed that the overall effect of social connections reported previously inversely equates with risk associated with social deficits, but it is presently unclear whether the deleterious effects of social deficits outweigh the salubrious effects of social connections. Currently, no meta-analyses focused on social isolation and loneliness exist in which mortality is the outcome. With efforts underway to identify groups at risk and to intervene to reduce that risk, it is important to understand the relative influence of social isolation and loneliness.
------
喫煙、座りがちな生活、大気汚染など、いくつかの生活習慣や環境要因が早期死亡の危険因子である。しかし、科学的な文献では、死亡リスクに同等以上の影響を与えることが証明された社会的要因にはあまり注意が払われていない(Holt-Lunstad, Smith, & Layton, 2010)。社会的なつながりを持つことは、心理的・感情的な幸福に影響を与えるだけでなく、身体的な幸福(内野、2006)および全体的な長寿(Holt-Lunstadら、2010; House, Landis, & Umberson, 1988; Shor, Roelfs, & Yogev, 2013)に有意かつプラスの影響を与えるのである。社会的つながりの欠如は、これまでの研究でも、有害な健康アウトカムに関連している。社会的関係の広範な保護効果は知られているが、このメタ分析レビューでは、社会的欠陥に関連するリスク上昇を支持する証拠に対する研究者の理解を狭めることを目的としている。具体的には、研究者は、これまでに報告された社会的つながりの全体的な効果が、社会的欠陥に関連するリスクと逆相関すると仮定してきたが、社会的欠陥の有害な効果が社会的つながりの有益な効果を上回っているかどうかは、今のところ不明である。現在、社会的孤立と孤独に焦点を当てたメタアナリシスで、死亡率をアウトカムとしたものは存在しない。リスクのあるグループを特定し、そのリスクを減らすために介入する努力が行われている中で、社会的孤立と孤独の相対的な影響力を理解することは重要である。
------
Living alone, having few social network ties, and having infrequent social contact are all markers of social isolation. The common thread across these is an objective quantitative approach to establish a dearth of social contact and network size. Whereas social isolation can be an objectively quantifiable variable, loneliness is a subjective emotional state. Loneliness is the perception of social isolation, or the subjective experience of being lonely, and thus involves necessarily subjective measurement. Loneliness has also been described as the dissatisfaction with the discrepancy between desired and actual social relationships (Peplau & Perlman, 1982). Is there a need to distinguish between social isolation and loneliness in assessing mortality risk? People lacking human contact often feel lonely (Yildirim & Kocabiyik, 2010); however, social isolation and loneliness are often not significantly correlated (Coyle & Dugan, 2012; Perissinotto & Covinsky, 2014), suggesting that these may be independent constructs and that one may occur without the other. For instance, some may be socially isolated but content with minimal social contact or actually prefer to be alone; others may have frequent social contact but still feel lonely. Because of the conceptual distinction between social isolation and loneliness, understanding their relative influence on mortality may provide insights into possible independent pathways by which each influences risk and, in turn, guides intervention efforts.
------
一人暮らし、社会的ネットワークのつながりの少なさ、社会的接触の頻度の少なさなどは、すべて社会的孤立の指標となります。これらに共通するのは、社会的接触の少なさやネットワークの大きさを客観的に定量的に立証することです。社会的孤立が客観的に定量化できる変数であるのに対して、孤独は主観的な感情状態です。孤独は社会的孤立の認識、つまり孤独であるという主観的な経験であり、したがって必然的に主観的な測定が必要となります。また、孤独は、望ましい社会的関係と実際の社会的関係の間の不一致に対する不満とも言われています(Peplau & Perlman, 1982)。死亡リスクの評価において、社会的孤立と孤独を区別する必要があるのでしょうか?人との接触がない人はしばしば孤独を感じるが(Yildirim & Kocabiyik, 2010)、社会的孤立と孤独はしばしば有意に相関しない(Coyle & Dugan, 2012; Perissinotto & Covinsky, 2014)ため、これらは独立した構成要素であり、一方がなくても発生する可能性があることが示唆される。例えば、社会的に孤立していても、最低限の社会的接触で満足していたり、実際に一人でいることを好む人もいれば、頻繁に社会的接触があっても、孤独を感じる人もいます。社会的孤立と孤独は概念的に区別されるので、死亡率に対するこれらの相対的な影響を理解することは、それぞれがリスクに影響を与え、ひいては介入努力の指針となる独立した経路の可能性について洞察を与えるかもしれない。
------
There are several processes by which actual and perceived social isolation may influence mortality risk (also see other reviews in this special section). Social connections, or the lack thereof, can influence health and risk of mortality via direct and indirect pathways (see Uchino, 2006). Both loneliness and social isolation are associated with poorer health behaviors including smoking, physical inactivity, and poorer sleep (Cacioppo et al., 2002; Hawkley, Thisted, & Cacioppo, 2009; Theeke, 2010). Each is also associated with health-relevant biological processes, including higher blood pressure, C-reactive protein, lipid profiles, and poorer immune functioning (Grant, Hamer, & Steptoe, 2009; Hawkley & Cacioppo, 2010; Pressman et al., 2005). Researchers that have included both social isolation and loneliness have linked these factors independently to poorer health behaviors and biological risk factors (Pressman et al., 2005; Shankar, McMunn, Banks, & Steptoe, 2011). However, few researchers have examined these concurrently, and little is known about their relative or synergistic influence. In this meta-analytic review, our primary aim was to focus on the relative effects of objective and subjective social isolation on mortality (the likelihood of death over a given time), to determine the magnitude and nature of the association with risk of mortality, and to identify potential moderating variables. We reviewed studies of mortality that included measures of loneliness, social isolation, or living alone. Because it is important to determine the effect of social isolation and loneliness independent of correlated lifestyle (e.g., smoking, physical activity) and psychological factors (e.g., depression, anxiety), we also examined inclusion of covariates.
------
実際の社会的孤立や社会的孤立の認知が死亡率に影響を与えるプロセスはいくつかある(本特集の他のレビューも参照のこと)。社会的なつながり、あるいはその欠如は直接的、間接的な経路で健康や死亡リスクに影響を与える可能性がある(Uchino, 2006参照)。孤独と社会的孤立はともに、喫煙、運動不足、睡眠不足など、より悪い健康行動と関連している (Cacioppo et al., 2002; Hawkley, Thisted, & Cacioppo, 2009; Theeke, 2010)。また、血圧の上昇、CRP、脂質プロファイル、免疫機能の低下など、健康に関連する生物学的過程とも関連している(Grant, Hamer, & Steptoe, 2009; Hawkley & Cacioppo, 2010; Pressman et al.) 社会的孤立と孤独の両方を含む研究者は、これらの要因をより悪い健康行動や生物学的危険因子と独立して結びつけています(Pressman et al.、2005;Shankar、McMunn、Banks、& Steptoe、2011)。しかし、これらを同時に検討した研究者は少なく、これらの相対的あるいは相乗的な影響についてはほとんど知られていない。このメタ分析レビューでは、死亡率(一定期間内に死亡する可能性)に対する客観的・主観的社会的孤立の相対的影響に焦点を当て、死亡リスクとの関連の大きさと性質を明らかにし、潜在的な緩和変数を特定することを主目的とした。我々は、孤独感、社会的孤立、一人暮らしの尺度を含む死亡率に関する研究をレビューした。社会的孤立や孤独の影響を、相関するライフスタイル(例:喫煙、身体活動)や心理的要因(例:うつ病、不安)から独立して判断することが重要であるため、共変数を含むかどうかも検討した。
------
Method
方法
------
Identification of studies
研究の特定
------
We identified published and unpublished studies of the association between social relationships and mortality using two techniques. First, we searched for studies appearing from January 1980 to February 2014 using several electronic databases: MEDLINE, CINAHL, PsycINFO, Social Work Abstracts, and Google Scholar. To capture relevant articles, we used multiple search terms, including mortality, death, decease(d), died, dead, and remain(ed) alive, which were crossed with synonyms of the terms social isolation, loneliness, and living alone. To minimize inadvertent omissions, we searched each database twice, with searches ending on February 24, 2014. Second, we manually examined the reference sections of past reviews and of studies meeting the inclusion criteria to locate articles not identified in the database searches. A team of research assistants who were trained and supervised by the authors conducted the searches.
------
社会的関係と死亡率の関連について、2つの手法で公表済みおよび未公表の研究を同定した。まず、1980年1月から2014年2月までに出現した研究を、いくつかの電子データベースを用いて検索した。MEDLINE、CINAHL、PsycINFO、Social Work Abstracts、およびGoogle Scholarである。関連する論文を捉えるために、mortality, death, decease(d), died, dead, remain(ed) aliveなどの複数の検索語を用い、social isolation, loneliness, living aloneの同義語と掛け合わせた。不用意な脱落を最小限にするため、各データベースを2回検索し、検索は2014年2月24日に終了した。次に,データベース検索で特定されなかった論文を見つけるために,過去のレビューや包括基準を満たした研究の参考文献のセクションを手作業で調査した。検索は,著者らによって訓練・指導されたリサーチアシスタントのチームが行った。
------
Inclusion criteria
対象基準
------
We included in the meta-analysis studies written in English that provided quantitative data regarding individuals’ mortality as a function of objective and subjective social isolation (operational definitions of social isolation, loneliness, and living alone are provided in Table 1). All studies needed to be prospective in design, meaning that the researchers measured one’s objective or subjective social isolation at the study initiation and then followed participants over time (typically several years) to determine who remained alive and who was dead at the follow-up. Thus, risk for mortality is an estimate of the extent to which social isolation, living alone, and loneliness significantly predict the likelihood of being dead at follow-up. We extracted data when authors used measures including the terms found in Table 1. In some cases, authors operationalized social isolation by contrasting the participants from the bottom quartile or quintile on a social network or integration measure (e.g., Social Network Index; Cohen, Doyle, Skoner, Rabin, & Gwaltney, 1997) but otherwise did not code data from measures of social networks/integration. Because we were interested in the impact of social deficits on disease, we excluded studies in which mortality was a result of suicide or accident. We also excluded studies in which the outcome could not be isolated to mortality (e.g., combined outcomes of morbidity and mortality). Although we excluded single-case designs and reports with exclusively aggregated data (e.g., census-level statistics), we included all other types of quantitative research designs that yielded a statistical estimate of the association between mortality and loneliness/isolation. Figure 1 shows the flow diagram containing the details of study inclusion (included in the Supplemental Material available online).
------
我々は、客観的・主観的社会的孤立の機能として個人の死亡率に関する定量的データを提供する英語で書かれた研究をメタ分析の対象とした(社会的孤立、孤独、一人暮らしの運用上の定義は表1に示されている)。すべての研究はプロスペクティブデザインである必要があり、研究者は研究開始時に客観的または主観的社会的孤立を測定し、その後参加者を長期間(通常数年間)追跡調査し、追跡調査時に誰が生存していて誰が死亡しているかを決定したことを意味します。したがって、死亡リスクとは、社会的孤立、一人暮らし、孤独が、追跡調査時に死亡している可能性をどの程度有意に予測するかを推定するものである。我々は、著者が表1にある用語を含む測定法を用いている場合にデータを抽出した。場合によっては、社会的ネットワークや統合の指標(例えば、Social Network Index; Cohen, Doyle, Skoner, Rabin, & Gwaltney, 1997)の下位4分の1または5分の1の参加者と対比することで社会的孤立を運用したが、それ以外は社会的ネットワーク/統合の指標からデータをコード化しなかった。我々は、社会的欠陥が疾病に及ぼす影響に関心があったので、死亡が自殺または事故の結果である研究は除外した。また、アウトカムが死亡率に分離できない研究(例えば、罹患率と死亡率の複合アウトカム)も除外した。シングルケースデザインと集計データのみの報告(例:国勢調査レベルの統計)は除外したが、死亡率と孤独・孤立の関連性を統計的に推定できるその他のタイプの定量的研究デザインはすべて対象とした。図 1 は、研究の組み入れの詳細を含むフロー図である(オンラインで利用可能な補足資料に含まれる)。
------
Data abstraction
データの抽象化
------
A team of research assistants and the authors performed the data searches and coding. To reduce the likelihood of human error in coding, a team of two raters coded each article twice. Two different raters performed the second coding of each article. Thus, two distinct coding teams (four raters) coded each article. Coders extracted several objectively verifiable characteristics of the studies: (a) the number of participants and their composition by age, gender, health status, and preexisting health conditions (if any), as well as the cause of mortality; (b) length of follow-up; (c) research design; (d) type of social isolation (actual/perceived) evaluated; (e) number and class of covariates included in the statistical model; and (f) exclusion of participants who were severely ill or who died shortly after study initiation. The latter two variables helped to address possible confounds (e.g., depression, health status, physical mobility, age) and reverse causality, whereby individuals with impaired health would be more likely to report increased social isolation or loneliness because of an inability to engage in social contact. For each study, we extracted the reported effect size, making sure that odds ratio (OR) values greater than one represented an increase in mortality as a function of social isolation, loneliness, or living alone?and a decrease in mortality when individuals were not isolated, lonely, or living alone. Effect sizes less than one indicated the opposite. To analyze the data, we temporarily transformed the reported effect sizes to the natural log of the OR and subsequently transformed them back to ORs for purposes of interpretation. When researchers reported multiple effect sizes within a study at the same point in time, we averaged the several values (weighted by standard error) to avoid violating the assumption of independent samples. We therefore used the shifting units of analysis approach (Cooper, 1998), which minimizes the threat of nonindependence in the data while allowing for more detailed follow-up analyses. In a few cases in which researchers reported multiple effect sizes across different levels of social isolation (high vs. medium, medium vs. low), we extracted only the value with the greatest contrast (high vs. low).
------
データ検索とコーディングは、リサーチアシスタントと著者のチームが行った。コーディングにおけるヒューマンエラーの可能性を減らすため、2 人の評価者チームが各論文を 2 回コーディン グした。各論文の 2 回目のコーディングは、異なる 2 名の評価者が行った。このように、2 つの異なるコーディングチーム(4 名の評価者)が各記事をコーディン グした。(a)参加者数、年齢、性別、健康状態、既往症(ある場合)、および死亡原因による構成、(b)追跡期間の長さ、(c)研究デザイン、(d)評価した社会的孤立(実際/知覚)の種類、(e)統計モデルに含めた共変量の数および種類、(f) 重病または研究開始後すぐに死亡した参加者の除外など、コーダーは研究の客観的検証を行ういくつかの特性を抜き出しました。後者の2つの変数は、起こりうる交絡因子(例:うつ病、健康状態、身体的移動性、年齢)および逆因果(健康障害のある人は、社会的接触ができないために社会的孤立や孤独の増加を報告する可能性が高い)に対処するのに役立つものである。各研究について、報告された効果量を抽出し、1より大きいオッズ比(OR)値が、社会的孤立、孤独、または一人暮らしの機能として死亡率の増加を表し、個人が孤立、孤独、または一人暮らしではない場合に死亡率が減少することを確認する。1未満の効果量は、その逆を表していた。データを分析するために、報告された効果量を一時的にORの自然対数に変換し、その後、解釈のためにORに変換しなおした。研究者が同じ時点の研究内で複数の効果量を報告した場合、独立標本の仮定に違反しないように、複数の値を平均化した(標準誤差で重み付け)。そのため、データにおける非独立性の脅威を最小限に抑えつつ、より詳細な追跡分析が可能なshifting units of analysis approach (Cooper, 1998)を使用した。研究者が異なる社会的孤立のレベル(高対中、中対低)で複数の効果量を報告しているいくつかのケースでは、最も対照的な値(高対低)のみを抽出した。
------
時間軸で複数の効果量を持つ研究がある場合、最も長い追跡期間のデータを抽出した。未調整のデータと、統計的コントロールの数が最も多いモデルからのデータを抽出した(ただし、両モデルで使用した統計的コントロールの種類と数を記録した後、その後の比較のために、最も少ない統計的コントロールを用いたモデルからのデータも抽出した)。全体として、データ抽出のための評価者間の一致は、カテゴリー変数(Cohenのカッパは平均0.73)および連続変数(単一の測定のクラス内相関は平均0.95)については十分に高いものであった。コーディングチーム間の不一致は、コンセンサスが得られるまで論文をさらに精査して解決した。
------
When a study contained multiple effect sizes across time, we extracted the data from the longest follow-up period. We extracted both unadjusted data and the data from the model involving the greatest number of statistical controls (although we also extracted the data from the model utilizing the fewest number of statistical controls for a subsequent comparison after recording the type and number of statistical controls used within both models). Overall, the interrater agreement for data abstraction was adequately high for categorical variables (with Cohen’s kappa averaging .73) and for continuous variables (with intraclass correlations for single measures averaging .95). We resolved discrepancies across coding teams through further scrutiny of the article until we obtained consensus.?
------
Results
成果
Description of the retrieved literature
検索した文献の説明
We located 79 articles reporting pertinent data, 9 of which were excluded because they contained the same data as another article, resulting in 70 independent studies that met the full inclusion criteria. The complete list of references and a table summarizing the characteristics of those studies (Table S1) are found in the Supplemental Material available online. Studies typically involved older adults, with a mean age of 66.0 years at initial data collection and with a mean length of follow-up being 7.1 years. Most studies (63%) involved normal community samples, but 37% of studies involved patients with a medical condition, such as heart disease. See Table 2 for further descriptive data. Three studies included data on both loneliness and one of the objective independent variables: two for loneliness and social isolation, and one for loneliness and living alone. Using a shifting units of analysis approach (Cooper, 1998), we included data from those distinct measures in the analyses specific to the type of measurement, but all other studies contributed a single data point to the analyses.
------
関連データを報告した79件の論文を探し出し、そのうち9件は他の論文と同じデータを含んでいたため除外し、その結果、完全な組み入れ基準を満たした独立した70件の研究が得られた。文献の完全なリストと、これらの研究の特徴をまとめた表(表S1)は、オンラインで利用可能な補足資料に記載されている。研究は一般的に高齢者を対象としており、最初のデータ収集時の平均年齢は66.0歳で、平均追跡期間は7.1年であった。ほとんどの研究(63%)は通常の地域住民を対象としているが、37%の研究は心臓病などの病状を持つ患者を対象としている。記述的なデータについては表2を参照。3つの研究では、孤独と客観的な独立変数の両方に関するデータを含んでいた。2つは孤独と社会的孤立、1つは孤独と一人暮らしである。分析単位の移行アプローチ(Cooper, 1998)を用いて、我々は、測定のタイプに特有の分析にこれらの異なる測定からのデータを含めましたが、他のすべての研究は、分析に単一のデータポイントを提供しました。
------
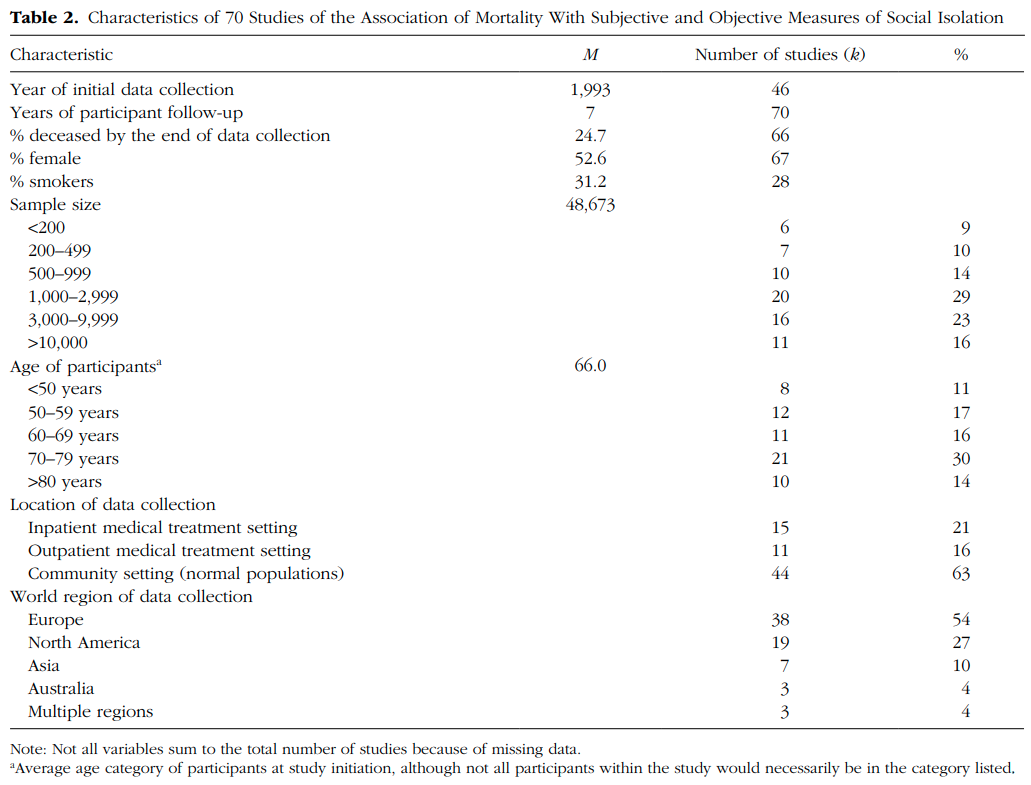
Effect sizes in the 70 studies had been calculated by researchers using a variety of methods, with some researchers reporting unadjusted values and with other researchers using a variety of covariates. ORs ranged from 0.64 to 3.85, with exceptionally high heterogeneity across studies (I 2 = 97.8%, 95% CI [97.6%, 98.1%]; Q = 3,328.9, p < .0001), suggesting excessive variability in findings across all types of data. We therefore divided the analyses according to the number of covariates used. In the unadjusted data group, the researchers controlled for no other variables in the analyses. In the partially adjusted data group, the researchers typically controlled for one or two variables, usually age and gender. The fully adjusted data are the model within studies with the largest number of covariates. Effect sizes from each category were evaluated separately, such that a single study could contribute effect sizes to more than one category (see Table 3).
------
70件の研究の効果量は、研究者によってさまざまな方法で計算されており、ある研究者は未調整値を報告し、他の研究者はさまざまな共変数を使用していた。ORは0.64から3.85の範囲で、研究間の異質性は例外的に高く(I 2 = 97.8%, 95% CI [97.6%, 98.1%]; Q = 3,328.9, p < .0001)、すべての種類のデータで所見が過度に変動していることが示唆された。そこで、使用した共変量の数によって解析を分けた。未調整データ群では、研究者は分析において他の変数を一切コントロールしなかった。部分調整データ群では、研究者は通常1つか2つの変数、通常は年齢と性別をコントロールした。完全調整データは、共変量が最も多い研究内のモデルである。各カテゴリーの効果量は別々に評価され、1つの研究が2つ以上のカテゴリーに効果量を寄与することもある(表3参照)。
------
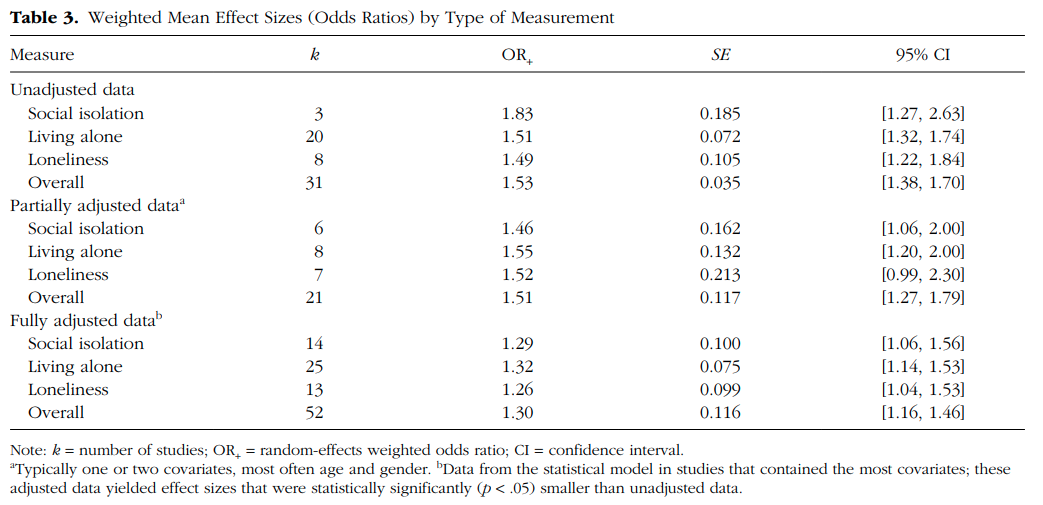
------
Overall, each of the measures (social isolation, loneliness, and living alone) for each type of data (unadjusted, partially adjusted, or fully adjusted) had an OR between 1.26 and 1.83. The three measures did not differ in their ORs for any of the three types of data, meaning that there was no overall difference among the two objective and one subjective factors. (Random-effects weighted analyses of variance across the measures yielded all ps > .20.) However, the type of data did matter in the analysis. Unadjusted data yielded effect sizes of greater magnitude than fully adjusted data (see Table 3). The differences between unadjusted and fully adjusted data also reached statistical significance (p < .001) when comparing data within 27 studies in which researchers reported more than one statistical model (e.g., unadjusted compared with fully adjusted values) using multivariate meta-analytic methods after accounting for the .74 correlation of effect sizes within studies. Thus, unadjusted and fully adjusted data not only represented conceptually distinct classes of data but also yielded findings of different magnitude.
------
全体として、各データタイプ(未調整、部分調整、完全調整)の各測定値(社会的孤立、孤独、一人暮らし)のORは1.26から1.83の間であった。3つの尺度は、3種類のデータのいずれにおいてもORに差がなく、2つの客観的要因と1つの主観的要因の間に全体的な差がないことを意味している。(尺度間のランダム効果による重み付け分散分析では、すべてのpsが.20以上であった)。しかし、データの種類は分析に重要であった。未調整のデータは、完全調整のデータよりも大きな効果量を示した(表3参照)。未調整データと完全調整データの差は、研究者たちが2つ以上の統計モデル(例えば、完全調整値と未調整値)を報告した27の研究内のデータを、研究内の効果量の相関0.74を考慮した後に多変量メタ分析法を用いて比較した場合にも、統計的に有意(p < 0.001)に達した。このように、未調整データと完全調整データは、概念的に異なるクラスのデータを表すだけでなく、異なる大きさの所見をもたらすものであった。
------
Moderator analyses
Given the substantial heterogeneity of the overall results (I 2 > 80%), we analyzed the extent to which the variability in effect sizes could be attributable to study or participant characteristics. These analyses involved only the fully adjusted data because multiple factors predictive of mortality had been controlled (thus minimizing possible confounding explanations). Study and participant characteristics included both categorical and continuous data, so we report those analyses separately.
------
モデレータ分析
全体的な結果のかなりの異質性(I 2 > 80%)を考慮して、効果量の変動が研究または参加者の特徴にどの程度まで起因しうるかを分析した。これらの解析は、死亡率を予測する複数の要因がコントロールされていたため、完全に調整されたデータのみを対象とした(したがって、交絡する可能性のある説明は最小限に抑えられている)。研究および参加者の特性には、カテゴリーデータおよび連続データの両方が含まれるため、これらの分析を別々に報告する。
------
Categorical variables.
We examined categorical variables using random-effects weighted analyses of variance, beginning with the type of covariates used in the fully adjusted models. Eight studies included multiple covariates that were directly relevant to social support, such as marital status, social networks, and loneliness. These eight studies had lower averaged effect sizes (OR = 1.17) than those of 33 studies in which no covariates directly relevant to social support were included in the statistical model (OR = 1.27). Otherwise, the averaged effect sizes remained of similar magnitude irrespective of the particular covariates that were or were not included in the models (p > .20), including covariates relevant to depression, socioeconomic status, health status, physical activity, smoking, gender, and age. Different combinations of covariates across studies yielded similar results. We found no substantive differences in effect sizes (p > .15) across the other categorical variables evaluated: world region, data collection setting, cause of mortality, research design, health status, and medical condition at intake. Finding no significant differences across participant health status when using the fully adjusted data was particularly notable because of a difference that we observed with the unadjusted data: Studies in which participants had a medical condition and were recruited from a medical setting had larger unadjusted average effect sizes (OR = 1.82) than studies with ostensibly healthy participants recruited from the general community (OR = 1.34, p = .003). Furthermore, with the unadjusted data, studies in which the researchers excluded participants with terminal conditions or participants who died shortly after baseline data collection (whose social isolation or social support may have been affected by their medical condition) had higher averaged effect sizes (OR = 1.95) than the studies in which the researchers did not report such exclusions (OR = 1.38, p < .05). Thus, accounting for participants’ initial health condition in the research design resulted in systematically different findings across studies. In most (81%) of the multivariate statistical models, researchers had controlled for participant health status variables, such that we found no differences across those conditions in the fully adjusted data. Studies in which the researchers controlled for health status variables yielded substantially different findings from those studies in which this was not done.
------
カテゴリー変数
完全調整モデルで使用した共変量の種類から、ランダム効果加重分散分析を使ってカテゴリー変数を検討した。8件の研究では、配偶者の有無、社会的ネットワーク、孤独感など、社会的支援に直接関連する複数の共変量が含まれていた。これらの8件の研究では、統計モデルに社会的支援に直接関連する共変量が含まれていない33件の研究(OR = 1.27)よりも、平均した効果の大きさが小さかった(OR = 1.17)。それ以外では、うつ病、社会経済的状態、健康状態、身体活動、喫煙、性別、および年齢に関連する共変量を含め、モデルに含めたり含めなかったりした特定の共変量に関係なく(p > .20)、平均した効果サイズは同じ大きさのままであった。研究間で共変量の組み合わせが異なっても、同様の結果が得られた。評価した他のカテゴリー変数(世界の地域、データ収集設定、死亡原因、研究デザイン、健康状態、摂取時の病状)間の効果量に実質的な差は認められなかった(p > 0.15)。完全に調整されたデータを使用しても、参加者の健康状態に有意差がないことは、未調整のデータで観察された違いのため、特に注目された。参加者が医学的状態にあり、医療環境から募集された研究では、一般社会から募集された表向きは健康な参加者の研究(OR = 1.34, p = .003)よりも、未調整の平均効果サイズが大きかった(OR = 1.82). さらに、未調整のデータでは、研究者が末期状態の参加者またはベースラインのデータ収集直後に死亡した参加者(その社会的孤立または社会的支援が病状に影響されたかもしれない)を除外した研究は、研究者がそのような除外を報告しなかった研究(OR = 1.38、p < .05)よりも平均効果量が大きかった(OR = 1.95)。このように、研究デザインにおいて参加者の最初の健康状態を考慮することで、研究間で系統的に異なる知見が得られた。多変量統計モデルのほとんど(81%)で、研究者は参加者の健康状態変数を制御しており、完全に調整されたデータでは、それらの条件による差は認められなかった。研究者が健康状態変数を制御した研究は、制御していない研究と実質的に異なる結果をもたらした。
------
Continuous variables.
We examined study and participant characteristics involving continuous data in relation to the observed effect sizes using random-effects weighted regression coefficients (meta-regression). We observed no coefficients greater than the absolute value of .20 between effect sizes and the year of initial data collection, the length of follow-up, or the percentage of female participants in each study. However, the number of covariates included in multivariate models was moderately associated with effect size (r = ?.27). Visual inspection of the corresponding scatter plot indicated that when studies included seven or more covariates, effect sizes tended to be more homogeneous, without extremely high values. To clarify, the inclusion of many covariates did not substantively reduce the magnitude of the general findings, which tended to remain in the range of OR = 1.20?1.40, but it did eliminate all OR values greater than 1.66. Analyses also indicated that the association between the effect size and the average age of participants at intake was of a moderately strong magnitude (r = ?.34 for adjusted data, and r = ?.46 for unadjusted data). This association with participant age remained of the same magnitude when accounting for length of study followup (and participants’ age at the end of the study) and when age was or was not used as a statistical covariate. Examination of the scatter plot and breaking down the data into three approximately equal categories of initial participant age helped to interpret the correlation: Studies involving participants of an average age less than 65 years had an average effect size of OR = 1.57 for adjusted data, and OR = 1.92 for unadjusted data; studies involving participants of an average age between 65 and 75 years had an average effect size of OR = 1.25 for adjusted data, and OR = 1.32 for unadjusted data; and studies involving participants of an average age greater than 75 years had an average effect size of OR = 1.14 for adjusted data, and OR = 1.28 for unadjusted data. Adults less than 65 years of age appeared to be at greater risk of mortality when they lived alone or were lonely compared with older individuals in those same conditions, even after controlling for the effect of age and other covariates on mortality
------
連続変数。
連続的なデータを含む研究および参加者の特徴を、観察された効果量との関係において、ランダム効果で重み付けした回帰係数を用いて調べた(メタ回帰)。効果量と、最初のデータ収集年、追跡期間、または各研究における女性参加者の割合との間に、絶対値0.20を超える係数は観察されなかった。しかし、多変量解析モデルに含まれる共変量の数は、効果量と中程度の関連があった(r = ?27)。散布図を見ると、7つ以上の共変量が含まれている場合、効果量はより均質になる傾向があり、極端に高い値にはならないことがわかる。明確には、多くの共変量が含まれていても、一般的な知見の大きさは実質的に減少せず、OR = 1.20?1.40の範囲に留まる傾向があったが、1.66より大きいOR値はすべて排除された。また、効果量と摂取時の参加者の平均年齢との関連は、中程度の強さであることも示された(調整済みデータではr = ?34、未調整のデータではr = ?46)。この参加者年齢との関連は、研究追跡期間(および研究終了時の参加者の年齢)を考慮しても、また年齢を統計的共変量として用いた場合と用いなかった場合でも、同じ大きさであった。散布図を調べ、データを参加者の初期年齢をほぼ等しい3つのカテゴリーに分類することで、相関を解釈することができました。平均年齢65歳未満の参加者を含む研究の平均効果量は、調整済みデータでOR = 1.57、未調整データでOR = 1.92だった;平均年齢65~75歳の参加者を含む研究の平均効果量は、調整済みデータでOR = 1.25、未調整データでOR = 1.32だった;平均年齢75歳超の参加者を含む研究は平均効果量が調整済みデータでOR = 1.14、未調整データでOR = 1.28 であった。65歳未満の成人は、死亡率に対する年齢や他の共変量の影響を制御した後でも、同じ条件の高齢者と比較して、一人暮らしや孤独である場合に死亡リスクが高いようであった。
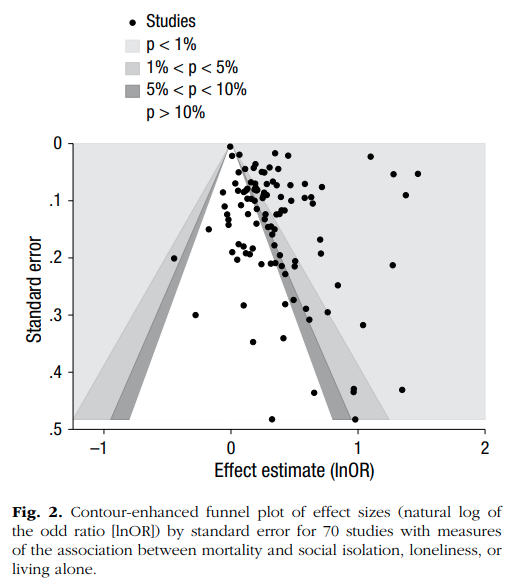
Fig. 2. Contour-enhanced funnel plot of effect sizes (natural log of the odd ratio [lnOR]) by standard error for 70 studies with measures of the association between mortality and social isolation, loneliness, or living alone.
図2. 死亡率と社会的孤立、孤独、または一人暮らしとの関連を測定した70の研究の標準誤差による効果量(奇数比の自然対数[lnOR])の輪郭強調ファネルプロット(Contour-enhanced funnel plot)。
------
Likelihood of publication bias adversely influencing the results
Publication bias occurs when the data obtained in a meta-analysis fail to represent the entire population of studies because of the increased probability of nonsignificant results remaining unpublished (and therefore less accessible for meta-analytic reviews). As can be seen in Figure 2, the data in this meta-analysis were highly variable, and the distribution of effect sizes appeared somewhat imbalanced toward the right side of the graph. The distribution of the data was relatively sparse toward the bottom of the white-shaded center of the graph, the area of nonsignificance. This kind of distribution can suggest that some nonsignificant studies were missing from the meta-analysis. However, neither Egger’s regression test nor an alternative to that test recommended for OR data (Peters, Sutton, Jones, Abrams, & Rushton, 2006) reached statistical significance (p > .05), which diminished the likelihood of possible publication bias. We found the fail-safe N?the number of hypothetically missing studies needed to reduce the present results to zero? to be 1,268, a number higher than the plausible number of studies conducted. Furthermore, using the trim and fill method (Duval & Tweedie, 2000), we did not estimate any “missing” studies; the distribution was overall fairly symmetric relative to the average effect size. It thus seemed unlikely that publication bias substantively affected the results of this meta-analysis.
------
結果に悪影響を及ぼす出版バイアスの可能性
出版バイアスは、メタアナリシスで得られたデータが、有意でない結果が未発表のまま(つまりメタアナリシスのレビューにアクセスしにくい)残る可能性が高く、研究集団全体を代表していない場合に発生します。図2に見られるように、このメタアナリシスのデータは非常に多様であり、効果量の分布はグラフの右側に向かってやや不均衡に見える。また、グラフの中央の白い網掛け部分(無意図領域)の下側では、データの分布が比較的まばらであることがわかる。このような分布は、有意でない研究がメタ分析から抜け落ちていることを示唆する。しかし、Eggerの回帰検定も、ORデータに推奨されるその検定の代替検定(Peters, Sutton, Jones, Abrams, & Rushton, 2006)も統計的有意性(p > .05)に達しておらず、出版バイアスの可能性が低くなっています。フェイルセーフのN(現在の結果をゼロにするために必要な仮想的な欠落研究の数)は1,268であり、これは実施された研究のもっともらしい数よりも多いことが分かった。さらに、trim and fill法(Duval & Tweedie, 2000)を用いて、「欠落」した研究はないと推定した。したがって、出版バイアスがこのメタアナリシスの結果に実質的に影響する可能性は低いと思われた。
------
Discussion
Social isolation results in higher likelihood of mortality, whether measured objectively or subjectively. Cumulative data from 70 independent prospective studies, with 3,407,134 participants followed for an average of 7 years, revealed a significant effect of social isolation, loneliness, and living alone on odds of mortality. After accounting for multiple covariates, the increased likelihood of death was 26% for reported loneliness, 29% for social isolation, and 32% for living alone. These data indicated essentially no difference between objective and subjective measures of social isolation when predicting mortality. The prospective designs of these studies and the statistical models that controlled for initial health status (and several other potential confounds) provide evidence for the directionality of the effect. Although we cannot confirm causality, the data show that individuals who were socially isolated, lonely, or living alone at study initiation were more likely to be deceased at the follow-up, regardless of participants’ age or socioeconomic status, length of the follow-up, and type of covariates accounted for in the adjusted models. We caution scholars perusing the expanding research literature on the association of social isolation and loneliness with physical health against reliance on unadjusted data because those data fail to account for participant health status, a factor contributing to reverse causality (when individuals with impaired health report increased loneliness or social isolation because their health condition limits their social contacts). Averaged results with unadjusted data were of greater magnitude than the results from fully adjusted models (see Table 3), particularly when participants had a preexisting health condition and when physically ill participants were not excluded from the unadjusted analyses. In fully adjusted models accounting for health status and in studies with physically ill individuals removed from analyses (thus accounting for reverse causality), social isolation and loneliness remained predictive of mortality. Future researchers will need to confirm the hypothesis that when individuals are ill (and ostensibly needing support) their risk for mortality increases substantially when lacking social support.
------
考察
社会的孤立は、客観的に測定しても主観的に測定しても、死亡の可能性を高くする。70の独立した前向き研究、3,407,134人の参加者を平均7年間追跡調査した累積データから、社会的孤立、孤独、一人暮らしが死亡の確率に有意に影響することが明らかになった。複数の共変数を考慮した結果、死亡の可能性の増加は、報告された孤独感で26%、社会的孤立で29%、一人暮らしで32%であった。
これらのデータは、死亡率を予測する際に、社会的孤立の客観的尺度と主観的尺度の間に本質的な差がないことを示していた。これらの研究のプロスペクティブデザインと、初期の健康状態(および他のいくつかの潜在的な交絡因子)をコントロールした統計モデルは、効果の方向性を示す証拠となる。因果関係は確認できないが、データは、参加者の年齢や社会経済状態、追跡期間の長さ、調整モデルで考慮した共変量の種類にかかわらず、研究開始時に社会的に孤立していた人、孤独だった人、一人暮らしだった人が追跡調査時に死亡している確率が高いことを示している。我々は、社会的孤立および孤独と身体の健康との関連に関する拡大する研究文献を閲覧する学者に対し、未調整データに依存しないよう注意を促す。なぜなら、それらのデータは、逆因果の要因(健康状態に障害のある個人が、その健康状態が社会的接触を制限するために孤独または社会的孤立が増加したと報告する場合)を参加者の健康状態を考慮できないためである。未調整データによる平均結果は、完全調整モデルによる結果よりも大きかった(表3参照)。特に、参加者に既往症がある場合、および体調不良の参加者を未調整分析から除外しなかった場合は、その傾向が強かった。健康状態を考慮した完全調整モデルや、身体疾患を持つ人を分析から除外した研究(逆因果を考慮した)でも、社会的孤立と孤独は死亡率の予測因子であることに変わりはなかった。今後の研究者は、個人が病気であるとき(そして表向きは支援を必要としているとき)、社会的支援を欠くと死亡リスクが大幅に増加するという仮説を確認する必要がある。
------
Overall, the findings from this meta-analysis are consistent with prior evidence that has demonstrated higher survival rates for those who are more socially connected (Holt-Lunstad et al., 2010) and extend those findings by focusing specifically on measurement approaches that assess the relative absence of social connections. Notably, the present meta-analysis included more than double the number of studies and 10 times the number of participants compared with the previous meta-analysis. Thus, the field now has much stronger evidence that lacking social connections is detrimental to physical health. The average effect sizes identified in this meta-analysis were lower than those reported previously for measures of social networks (OR = 1.45, 95% CI [1.32, 1.59]) and social integration (OR = 1.52, 95% CI [1.36, 1.69]) and were much lower than complex measures of social integration (OR = 1.91, 95% CI [1.63, 2.23]; see Table 4 of Holt-Lunstad et al., 2010). This difference may suggest that the salubrious effects of being socially connected may be stronger than the adverse effects of lacking connections. However, it is also likely that research methods that account for the multidimensionality of social relationships better predict mortality than measurement focused on any single aspect of sociality, such as social isolation. Nonetheless, identification of the relative effects of each component may be useful in targeting those that may be modifiable. There is also presently no research evidence to suggest a threshold effect. The aggregate results suggest more of a continuum than a threshold at which risk becomes pronounced. Although it is possible that individuals who are extremely lonely or socially isolated may account for much of the elevated risk, presently too few researchers target extremely isolated individuals in studies. Given the complexity (including objective and subjective aspects) of social relationships, identifying such a threshold seems unlikely.
------
全体として、このメタアナリシスから得られた知見は、社会的つながりが強い人ほど生存率が高いことを示した先行証拠と一致し(Holt-Lunstad et al.、2010)、社会的つながりの相対的欠如を評価する測定アプローチに特に焦点を当てることでこれらの知見を拡張している。注目すべきは、今回のメタアナリシスでは、前回のメタアナリシスと比較して、研究数が2倍以上、被験者数が10倍以上となっていることである。したがって、この分野では、社会的つながりの欠如が身体的健康に有害であることを示す、より強力な証拠が得られたのである。 このメタ分析で確認された平均効果量は、社会的ネットワーク(OR = 1.45, 95% CI [1.32, 1.59] )と社会的統合(OR = 1.52, 95% CI [1.36, 1.69] )については前回報告したものより低く、社会統合の複合指標よりはるかに低かった(OR = 1.91, 95% CI [1.63, 2.23]; Holt-Lunstad et al.の表4参照, 2010)。この違いは、社会的なつながりがあることの有益な効果は、つながりがないことの有害な効果よりも強いことを示唆しているのかもしれない。しかし、社会的関係の多次元性を考慮した調査方法の方が、社会的孤立のような社会性の単一側面に焦点を当てた測定よりも死亡率をより良く予測できる可能性もある。それでも、各要素の相対的な効果を明らかにすることは、修正可能な要素に的を絞る上で有用であろう。また、現在のところ、閾値効果を示唆する研究証拠もない。集計結果は、リスクが顕著になる閾値というより、連続性を示唆している。極端に孤独な人、社会的に孤立した人がリスクの上昇の多くを占める可能性はあるが、現在のところ、極端に孤立した人を研究対象としている研究者が少なすぎる。社会的関係の複雑さ(客観的、主観的側面を含む)を考慮すると、そのような閾値を特定することは不可能と思われる。
------
Objective versus subjective isolation
Using the meta-analytic data, had we found that either social isolation or loneliness was more predictive of mortality, interventions to reduce risk could have become more targeted. However, we presently have no evidence to suggest that one involves more risk than the other for mortality. Unfortunately, in the vast majority of studies, researchers examined only one measurement approach (social isolation, loneliness, or living alone), precluding direct comparisons. Among the few studies in which researchers contrasted social isolation and loneliness, the evidence was mixed, with researchers finding that loneliness was more influential in one study (Holwerda et al., 2012), and with other researchers finding that social isolation had stronger effects than loneliness in a later study (Steptoe, Shankar, Demakakos, & Wardle, 2013). This inconsistency may be due to differences in methodological approaches to handling correlated psychological states, such as depression (Booth, 2000). Our analyses indicated that the elevated risk of mortality persisted even when controlling for correlated components of social networks and multiple other factors, including depression, with the use of covariates negating large effect sizes. In any case, the multiple, overlapping components of sociality make reliance on statistical adjustment less desirable than direct comparisons between components, such as loneliness and social isolation. The equivalent effects of social isolation and loneliness reported here do not indicate interchangeability of these risk assessments. Rather, the available data suggest that efforts to mitigate risk should consider both social isolation and loneliness without the exclusion of the other. Because social isolation and loneliness are often weakly correlated (Coyle & Dugan, 2012), simply increasing social contact may not mitigate loneliness. Likewise, exclusively altering one’s subjective perceptions among those who remain objectively socially isolated may not mitigate risk.
------
客観的な孤立と主観的な孤立
メタ解析のデータを使って、もし社会的孤立と孤独のどちらかが死亡率をより予測することが分かっていれば、リスクを減らすための介入はより的を射たものになったかもしれない。しかし、現在のところ、死亡率に関してどちらか一方が他方よりもリスクが高いことを示す根拠はない。残念ながら、大半の研究では、研究者は1つの測定方法(社会的孤立、孤独、一人暮らし)だけを調べており、直接比較することはできない。研究者が社会的孤立と孤独を対比させた数少ない研究の中で、その証拠はまちまちで、ある研究では孤独の方がより影響力があるとし(Holwerda et al.、2012)、他の研究者は後の研究で社会的孤立が孤独より強い影響を与えるとした(Steptoe, Shankar, Demakakos, & Wardle, 2013)。この矛盾は、うつ病など相関のある心理状態を扱う方法論の違いによるものかもしれません(Booth, 2000)。我々の分析では、社会的ネットワークの相関成分やうつ病を含む他の複数の要因を制御しても死亡リスクの上昇は持続し、共変量の使用によって大きな効果量が否定されることが示された。いずれにせよ、社会性の構成要素が複数あり、重複しているため、統計的調整に頼ることは、孤独と社会的孤立のような構成要素間の直接比較よりも好ましくない。ここで報告された社会的孤立と孤独の同等の効果は、これらのリスク評価の互換性を示すものではない。むしろ、利用可能なデータは、リスクを軽減するための努力は、他方を排除することなく、社会的孤立と孤独の両方を考慮すべきであることを示唆している。社会的孤立と孤独はしばしば弱い相関があるため(Coyle & Dugan, 2012)、単に社会的接触を増やしても孤独は軽減されないかもしれない。同様に、客観的に社会的に孤立している人の主観的な認識を変えるだけでは、リスクは軽減されないかもしれない。
------
The evolutionary perspective of loneliness proposed by Cacioppo and colleagues (Cacioppo et al., 2006; Cacioppo, Cacioppo, & Boomsma, 2014) presents loneliness as an adaptive signal, similar to hunger and thirst, that motivates one to alter behavior in a way that will increase survival. Accordingly, loneliness is a powerful motivator to reconnect socially, which, in turn, increases survival and opportunity to pass on genes. Consistent with this perspective, intervention attempts to alter the signal (e.g., hunger, loneliness) without regard to the actual behavior (e.g., eating, social connection) and vice versa would likely be ineffective. Extending this possibility, some data have shown that those who are both high in loneliness and social isolation had the poorest immune response (Pressman et al., 2005). Therefore, both objective and subjective measures of social isolation should be considered in risk assessment. It is only through direct comparisons of social isolation and loneliness in the same sample that researchers can establish independent, relative, and synergistic effects. Consequently, it is possible that different combinations of social isolation and loneliness may represent different levels of risk. For instance, those low in both isolation and loneliness would presumably be at lowest risk, those high in both at highest risk, and those who are isolated but not lonely or lonely but not isolated to be at intermediate risk. Nonetheless, there is currently insufficient empirical evidence to test this hypothesis, highlighting an important weakness of the current literature that needs to be addressed in future research.
Cacioppoらが提唱する孤独の進化的視点(Cacioppo et al., 2006; Cacioppo, Cacioppo, & Boomsma, 2014)は、孤独を飢えや渇きと同様の適応的信号として示し、生存率を高めるように行動を変化させる動機付けとなるとしています。したがって、孤独は社会的なつながりを取り戻すための強力な動機づけとなり、その結果、生存率と遺伝子継承の機会が増加する。このような観点から、実際の行動(例:食事、社会的つながり)を無視してシグナル(例:空腹感、孤独感)を変える介入の試みは、おそらく効果がないだろう。この可能性を拡大すると、孤独感と社会的孤立感の両方が高い人は、免疫反応が最も悪いというデータもあります(Pressman et al.) 従って、リスク評価においては、社会的孤立の客観的、主観的な尺度の両方を考慮する必要があります。同じサンプルで社会的孤立と孤独を直接比較することによってのみ、研究者は独立した、相対的な、そして相乗的な効果を確立することができるのです。その結果、社会的孤立と孤独の異なる組み合わせが、異なるレベルのリスクを表している可能性があるのです。例えば、孤立と孤独の両方が低い人は最もリスクが低く、両方が高い人は最もリスクが高く、孤立しているが孤独ではない人、孤独だが孤立していない人は中間のリスクであると推測される。しかし、この仮説を検証する実証的証拠はまだ十分ではなく、現在の文献の重要な弱点が浮き彫りになっており、今後の研究で対処する必要がある。
------
Isolation and aging
The data in this meta-analysis should make researchers call into question the assumption that social isolation among older adults places them at greater risk compared with social isolation among younger adults. Using the aggregate data, we found the opposite to be the case. Middle-age adults were at greater risk of mortality when lonely or living alone than when older adults experienced those same circumstances. The moderating effect of age may seem counterintuitive in light of data indicating that individuals more than 65 years of age are more likely to report loneliness (Dykstra, van Tilburg, & de Jong Gierveld, 2005), but there are at least four plausible explanations for why middle-age adults may differ from older adults in terms of the relevance of social networks to physical health. First, it is possible that individuals who do not die early may be a particularly resilient group, with different social or health characteristics than those who die at earlier ages. Thus, the observed difference across age could be confounded with preexisting health status, although this interpretation is qualified by the fact that the researchers using multivariate statistical models accounted for participant age and health status. A second explanation involves changes in social networks as individuals transition from full-time employment to retirement, with decreases in socialization in occupational and public forums that are seen as culturally normative. This possible explanation is supported by one study in which researchers examined loneliness after retirement and found an effect for mental health (anxiety and depression) but not for physical health (functional status and number of chronic conditions; Bekhet & Zauszniewski, 2012). Third, it is plausible that individuals who are alone or lonely before retirement age may be more likely to engage in risky health behaviors or less likely to seek medical treatment early, whereas after retirement, people may attend more assiduously to their physical health.
------
孤立と老化
このメタ分析のデータは、高齢者の社会的孤立は若年者の社会的孤立よりもリスクが高いという仮定に疑問を投げかけるものである。集計データを用いて、我々はその逆であることを発見した。中年の成人は、高齢者が同じ状況を経験したときよりも、孤独または一人暮らしのときに死亡リスクが高くなることがわかった。 65歳以上の人は孤独を感じやすいというデータ(Dykstra, van Tilburg, & de Jong Gierveld, 2005)からすると、年齢の緩和効果は直感に反するように思われるが、身体の健康と社会ネットワークの関連性について、なぜ中年の成人が高齢者と異なるのかについては、少なくとも4つのもっともらしい説明がある。第一に、早期死亡しない人は、早期死亡する人とは異なる社会的特性や健康的特性を持つ、特に回復力の高い集団である可能性がある。したがって、年齢による違いは既存の健康状態と混同される可能性があるが、この解釈は、研究者が多変量統計モデルを用いて参加者の年齢と健康状態を説明したことによって限定されている。第二の説明は、常勤職から退職への移行に伴う社会的ネットワークの変化と、文化的に規範的とみなされる職業上および公的な場での社会化の減少が関係しているというものである。この説明は、退職後の孤独感を調査したある研究で支持されており、精神的健康(不安と抑うつ)には影響があったが、身体的健康(機能的状態や慢性疾患の数)には影響がなかったという。第三に、定年前に孤独であった人は、リスクの高い健康行動をとりやすい、あるいは早期に治療を受けにくいという可能性があり、一方、定年後は身体の健康により熱心に取り組むという可能性がある。
------
Finally, it is possible that the different results across participant age are confounded with marital status: Older adults are much more likely to be widows/widowers than middle-age adults. Our metaanalysis cannot shed light on these four possible explanations because the first three explanations involve variables inadequately evaluated in the present research literature, and the variable associated with the fourth explanation, marital status, was not coded in our analyses. Although many studies indicate that loneliness differs across marital status (Cacioppo & Patrick, 2008; Hughes et al., 2004; Victor & Bowling, 2012) and that marital status is significantly associated with mortality (Roelfs, Shor, Kalish, & Yogev, 2011), we did not include marital status as an indicator of social isolation because being unmarried does not necessarily mean that one is socially isolated, living alone, or lonely. Moreover, there would be multiple qualitative differences in the social networks of an older individual who had never been married compared with one who had been married and raised children but whose spouse had recently died, even though both are living alone. Rather than include all possibly correlated variables (e.g., marital status, depression, substance abuse), we evaluated only direct measures of social isolation, living alone, or loneliness. Given the limitations of the present meta-analysis, future researchers should confirm the apparent differences across participant age and should evaluate the relative merits of the several plausible explanations for that finding
------
最後に、参加者の年齢によって異なる結果は、配偶者の有無と交絡している可能性がある。高齢者は、中年者に比べて、寡婦・寡夫である可能性が非常に高い。最初の3つの説明は、現在の研究文献では十分に評価されていない変数が含まれており、4番目の説明である配偶者の有無に関連する変数は、我々の分析ではコード化されていないため、我々のメタ分析では、これらの4つの可能な説明に光を当てることができません。しかし、未婚であることが必ずしも社会的孤立、一人暮らし、孤独であることを意味しないため、社会的孤立の指標として未婚であることを含めないこととした。さらに、同じ一人暮らしでも、結婚歴のない高齢者と、結婚して子どもを育て、配偶者が最近亡くなった高齢者では、社会的ネットワークに質的な違いが複数存在すると思われる。相関のありそうな変数(例えば、配偶者の有無、うつ病、物質乱用)をすべて含めるのではなく、社会的孤立、一人暮らし、孤独の直接的尺度のみを評価した。今回のメタ分析の限界を考えると、今後の研究者は、参加者の年齢による明らかな差異を確認し、この発見に対するいくつかのもっともらしい説明の相対的な利点を評価する必要がある。
------
To better evaluate differences across age, future researchers should involve participants from a broad range of age groups. Most of the data in this meta-analysis came from older adults. Only 24% of studies involved people with an average age of 59 years or younger, and only 9% of studies involved people younger than 50 years of age at intake. If future data collection with younger adult samples confirms the age differences we observed in this meta-analysis, then widespread beliefs about the health risks of social isolation being greatest among older adults are inaccurate. In any case, the metaanalytic data, taken together with evidence for detrimental influences across the life span (Qualter et al., 2015, this issue), suggest that future research (and possibly interventions) should expand beyond older adults.
年齢による違いをより良く評価するために、今後の研究者は幅広い年齢層の被験者を参加させる必要がある。このメタ分析では、ほとんどのデータが高齢者から得られています。平均年齢59歳以下の人を対象とした研究は24%に過ぎず、摂取時年齢が50歳未満の人を対象とした研究は9%に過ぎない。もし、今後、若年層を対象にしたデータ収集で、このメタ分析で観察された年齢差が確認されれば、社会的孤立の健康リスクは高齢者で最も大きいという広く信じられていることは不正確であることになります。いずれにせよ、メタ分析データは、生涯にわたって有害な影響を及ぼす証拠(Qualterら、2015、本号)と合わせて考えると、今後の研究(そしておそらく介入)は、高齢者以外にも拡大すべきことを示唆している。
------
Conclusion
Substantial evidence now indicates that individuals lacking social connections (both objective and subjective social isolation) are at risk for premature mortality. The risk associated with social isolation and loneliness is comparable with well-established risk factors for mortality, including those identified by the U.S. Department of Health and Human Services (physical activity, obesity, substance abuse, responsible sexual behavior, mental health, injury and violence, environmental quality, immunization, and access to health care; see www.hhs.gov/safety/index). A substantial body of research has also elucidated the psychological, behavioral, and biological pathways by which social isolation and loneliness lead to poorer health and decreased longevity (for reviews, see Cacioppo, Cacioppo, Capitanio, & Cole, 2015, this issue; Shankar et al., 2011; Thoits, 2011; see also Cacioppo et al., 2015; Hawkley & Cacioppo, 2003, 2010). In light of mounting evidence that social isolation and loneliness are increasing in society (McPherson & Smith-Lovin, 2006; Perissinotto, Stijacic Cenzer, & Covinsky, 2012; Victor & Yang, 2012; Wilson & Moulton, 2010), it seems prudent to add social isolation and loneliness to lists of public health concerns. The professional literature and public health initiatives can accord social isolation and loneliness greater recognition.
------
結論
社会的なつながりのない人(客観的、主観的な社会的孤立)は早期死亡のリスクがあることを示す証拠は十分にある。社会的孤立や孤独に関連するリスクは、米国保健社会福祉省が特定したものを含む、確立された死亡の危険因子(身体活動、肥満、物質乱用、責任ある性行動、精神衛生、怪我と暴力、環境の質、予防接種、医療へのアクセス; www.hhs.gov/safety/index を参照)と同等である。また、多くの研究が、社会的孤立と孤独がより悪い健康と長寿の減少につながる心理的、行動的、生物学的経路を解明してきました(レビューとして、Cacioppo, Cacioppo, Capitanio, & Cole, 2015, this issue; Shankar et al.) 社会的孤立と孤独が社会で増加しているという証拠の積み重ねを考慮すると(McPherson & Smith-Lovin, 2006; Perissinotto, Stijacic Cenzer, & Covinsky, 2012; Victor & Yang, 2012; Wilson & Moulton, 2010)、公衆衛生に関するリストの中に社会的孤立と孤独を追加することは賢明であるように思われます。専門的な文献や公衆衛生の取り組みは、社会的孤立や孤独をより認識させることができます。
------
To draw a parallel, several decades ago scientists who observed widespread dietary and behavior changes (increasing consumption of processed and calorie-rich foods and increasingly sedentary lifestyles) raised warnings about obesity and related health problems (e.g., Brewster & Jacobson, 1978; Dietz & Gortmaker, 1985). The present obesity epidemic (Wang & Beydoun, 2007) had been predicted. Obesity now receives constant coverage in the media and in public health policy and initiatives. The current status of research on the risks of loneliness and social isolation is similar to that of research on obesity 3 decades ago?although further research on causal pathways is needed, researchers now know both the level of risk and the social trends suggestive of even greater risk in the future. Current evidence indicates that heightened risk for mortality from a lack of social relationships is greater than that from obesity (Flegal, Kit, Orpana, & Graubard, 2013; Holt-Lunstad et al., 2010), with the risk from social isolation and loneliness (controlling for multiple other factors) being equivalent to the risk associated with Grades 2 and 3 obesity. Affluent nations have the highest rates of individuals living alone since census data collection began and also likely have the highest rates in human history, with those rates projected to increase (e.g., Euromonitor International, 2014). In a recent report, researchers have predicted that loneliness will reach epidemic proportions by 2030 unless action is taken (Linehan et al., 2014). Although living alone can offer conveniences and advantages for an individual (Klinenberg, 2012), this meta-analysis indicates that physical health is not among them, particularly for adults younger than 65 years of age. Further research is needed to address the complexities of social interactions, interdependence, and isolation (Parigi & Henson, 2014; Perissinotto & Covinsky, 2014), but current evidence certainly justifies raising a warning.
------
数十年前、食生活や行動の変化(加工食品やカロリーの高い食品の消費の増加、座りがちなライフスタイルの増加)を観察した科学者たちは、肥満とそれに関連する健康問題について警告を発した(例:Brewster & Jacobson, 1978; Dietz & Gortmaker, 1985)。現在の肥満の流行(Wang & Beydoun, 2007)は予言されていた。現在、肥満は、メディアや公衆衛生政策、取り組みにおいて、常に取り上げられている。孤独と社会的孤立のリスクに関する研究の現状は、30年前の肥満に関する研究と似ている。因果関係の経路に関するさらなる研究が必要ではあるが、研究者は現在、リスクのレベルと将来的にさらにリスクが高まることを示唆する社会的傾向の両方を知っているのである。現在のエビデンスでは、社会的関係の欠如による死亡リスクの高まりは、肥満によるものよりも大きく、(Flegal, Kit, Orpana, & Graubard, 2013; Holt-Lunstad et al., 2010) 社会的孤立と孤独によるリスク(他の複数の要因を制御)は、グレード2および3の肥満に伴うリスクと同等であることが分かっています。豊かな国々は、国勢調査のデータ収集が始まって以来、一人暮らしの個人の割合が最も高く、また、人類史上最も高い割合であると考えられ、その割合は増加すると予測されています(例:Euromonitor International, 2014)。最近の報告書では、研究者は、対策を講じない限り、2030年までに孤独が伝染病の割合に達すると予測しています(Linehan et al.、2014年)。一人暮らしは個人にとって便利で利点がありますが(Klinenberg, 2012)、このメタ分析では、特に65歳未満の成人の場合、身体の健康はその中に含まれないことが示されています。社会的相互作用、相互依存、孤立の複雑さについてはさらなる研究が必要であるが(Parigi & Henson, 2014; Perissinotto & Covinsky, 2014)、現在のエビデンスは確かに警告を発するに値するものである。